In this tutorial, we will show how to develop Provider and Consumer web parts and connect them through an Interface.
The result will be the ability to have two web parts on a SharePoint 2010 page and filter the contents of the consumer web part on the data from the provider web part. This is almost like a master - detail view.
You can view the same functionality by looking at the standard SharePoint web parts. Modify a web part --> select Connections and the "Provide data to..." or "Receive data from..."
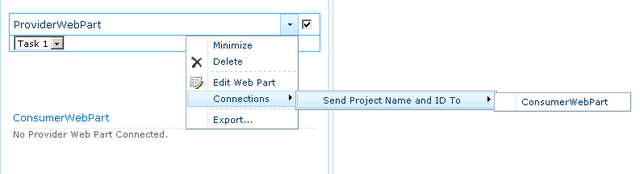
and then...
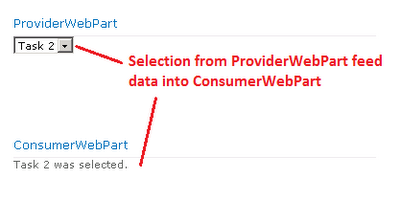
Lets Go:
We will show the detailed steps to:
1 - Develop a Connection Interface
2 - Develop a simple provider web part.
3 - Develop a simple consumer web part.
1 - Develop a Connection Interface.
Open Visual Studio 2010 and create a new project. In the New Project dialog window, select Visual C# --> SharePoint 2010 --> Empty SharePoint Project.
Provide a descriptive name and click on OK.
Select to deploy the solution as a "Deploy as Farm Solution" and click on Finish.
Wait for the solution to be created in Visual Studio.
Now we will create the web part connection interface which is responsible for exchanging connection information between a provider and consumer web part.
In the Solution Explorer, right-click on your project and select "Add --> New item".
In the Add New Item dialog window, select Visual C# --> Code --> Interface.
Enter ITaskin the Name textbox and click the Add button.
Open ITask.cs in code view and change the visibility of the interface to Public and add the following code inside the interface:
namespace WebPartConnectors
{
public interface ITask
{
int Id { get; }
string Name { get; }
}
}
2 - Develop a simple provider web part:
In the Solution Explorer, right click on your project and select Add --> New Item…
Select Visual C# --> SharePoint 2010 Web Part.
Enter ProviderWebPart in the Name textbox and click Add.
Open ProviderWebPart.cs in code view and in the ProviderWebPart class declaration, implement IProject.
public class ProviderWebPart : Microsoft.SharePoint.WebPartPages.WebPart, ITask
Insert the following code after the ProviderWebPart class declaration.
This code block implements the IProject web part connection interface and adds a local variable to the web part.
DropDownList _objPicker = null;
int ITask.Id
{
get
{
return int.Parse(_objPicker.SelectedValue);
}
}
string ITask.Name
{
get
{
return _objPicker .SelectedItem.ToString();
}
}
Update the CreateChildControls method to contain the following code:
protected override void CreateChildControls()
{
try
{
_objPicker= new DropDownList();
using (SPSite spSite = new SPSite(SPContext.Current.Web.Url))
using (SPWeb spWeb = spSite.OpenWeb())
{
SPList objList = spWeb.Lists["Tasks"];
foreach (SPListItem objListItem in objList.Items)
{
_objPicker.Items.Add(new ListItem(objListItem.Title, objListItem.ID.ToString()));
}
}
_objPicker.AutoPostBack = true;
this.Controls.Add(_objPicker);
}
catch (Exception ex)
{
this.Controls.Clear();
this.Controls.Add(new LiteralControl(ex.Message));
}
}
Insert the following ConnectionProvider property below the CreateChildControls method. This provides the Connection Provider interface point for the ProviderWebPart: [ConnectionProvider("Task Name and ID")]
public ITask NameDoesNotMatter()
{
return this;
}
Save your solution and build. Ensure that there are no build errors before you proceed.
3 - Develop a simple consumer web part.
In the Solution Explorer, right click on your project and select Add --> New Item…
Select Visual C# --> SharePoint 2010 Web Part.
Enter ConsumerWebPart in the Name textbox and click Add.
Insert the following code inside the ConsumerWebPart class declaration:
ITask _provider = null;
Label _lbl = null;
Update the CreateChildControls method to contain the following code:
protected override void CreateChildControls()
{
try
{
_lbl = new Label();
if (_provider != null)
{
if (_provider.Id > 0)
{
_lbl.Text = _provider.Name + " was selected.";
}
else
{
_lbl.Text = "Nothing was selected.";
}
}
else
{
_lbl.Text = "No Provider Web Part Connected.";
}
this.Controls.Add(_lbl);
}
catch (Exception ex)
{
this.Controls.Clear();
this.Controls.Add(new LiteralControl(ex.Message));
}
}
Insert the following ConnectionConsumer property below the CreateChildControls method. This provides the Connection Consumer interface point for the ConsumerWebPart:
[ConnectionConsumer("Name and ID")]Edit a page and add the two new web parts.
public void ThisNameDoesNotMatter(IProject providerInterface)
{
_provider = providerInterface;
}
Save the solution, Build the solution and Deploy the solution.
Go to your target SharePoint 2010 site and refresh the site.
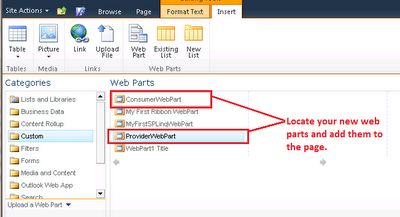
View your two new web parts on the page:
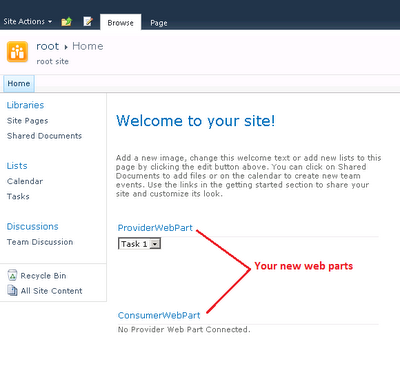
Edit the page again and select the ProviderWebPart and click on Edit Web Part.
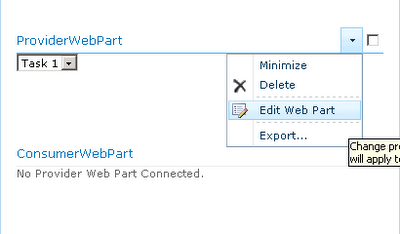
Select the ProviderWebPart again, but this time select "Connections" --> "Send Task Name and ID To" --> ConsumerWebPart.
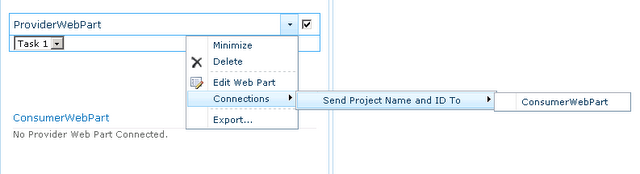
Now, if you select an item from the dropdown list control in the providerwebpart you will see the corresponding data change in the consumerwebpart.
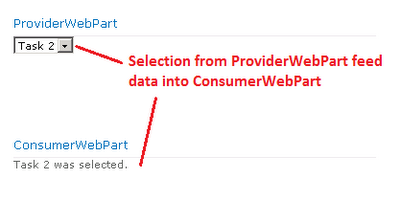
This way you will be able to build master-detail views or web part filters based on selections of other web parts
909091d5-0e04-4543-ab8b-bea2fac2c713|0|.0